Book notes: Tidy First?
Book notes on "Tidy First? A Personal Exercise in Empirical Software Design" by Kent Beck
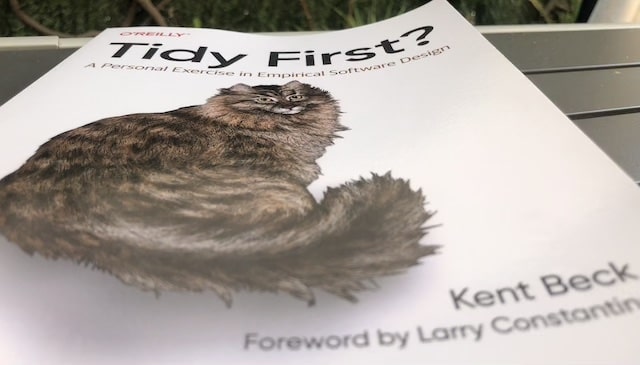
These are my notes on Tidy First? A Personal Exercise in Empirical Software Design by Kent Beck.
The book takes you from basic entry level refactorings to the economics of optionality, all in less than 100 pages.
Key Insights
- Coupling and cohesion are simply measures of complexity.
- SW design is an exercise in human relationships.
- Design some time around when you can take advantage of the design.
- Tidyings are a subset of refactoring that nobody could possibly hate on:
- Tidying is geek self-care.
- As a code reader, you expect that difference means difference.
- Sometimes better cohesion helps you live with the coupling.
- Done well, SW design enables SW design that enables change.
- Sometimes because of how small pieces interact, the code is harder to understand than one big pile of code.
- More than one hour tidying at a time before making any behavioral changes, likely means you have lost track of the minimum set of structural changes needed.
- Tidying later is a learning tool.
- Understanding theory optimizes application.
- SW designers can only:
- Create and delete elements.
- Create and delete relationships.
- Increase the benefit of a relationship.
- SW creates value in two ways:
- What it does today (== behaviour).
- The possibility of new things we can make it do tomorrow (== optionality).
- SW structure creates options.
- We can’t really tell if we have invested or invested enough in SW structure.
- Economic value of a SW system is the sum of the discounted future cash flows.
- Most SW design decisions are easily reversible, hence there is little value to avoiding mistakes, hence we shouldn’t invest much in doing so.
- Make decisions reversible.
- Analyzing coupling requires knowing what changes have happened and/or likely to happen.
- Cascading coupling are the bigger issue.
- Constantine’s equivalence:
- cost(sw) ~= cost(changes) ~= cost(big changes) ~= coupling.
- To reduce the cost of SW, we must reduce coupling.
- Make SW design an ordinary, balanced part of development.
- Tidy first? Likely yes. Just enough. You are worth it.
TOC
- Foreword
- Preface
- Introduction
- Part I - Tidyings
- Chapter 1 - Guard Clauses
- Chapter 2 - Dead Code
- Chapter 3 - Normalize Symmetries
- Chapter 4 - New Interface, Old Implementation
- Chapter 5 - Reading Order
- Chapter 6 - Cohesion Order
- Chapter 7 - Move Declaration and Initialization Together
- Chapter 8 - Explaining Variables
- Chapter 9 - Explaining Constants
- Chapter 10 - Explicit Parameters
- Chapter 11 - Chunk Statements
- Chapter 12 - Extract Helper
- Chapter 13 - One Pile
- Chapter 14 - Explaining Comments
- Part II - Managing
- Part III - Theory
- Chapter 22 - Beneficially Relating Elements
- Chapter 23 - Structure and Behaviour
- Chapter 24 - Economics: Time Value and Optionality
- Chapter 25 - A Dollar Today > A Dollar Tomorrow
- Chapter 26 - Options
- Chapter 27 - Options Versus Cash Flows
- Chapter 28 - Reversible Structure Changes
- Chapter 29 - Coupling
- Chapter 30 - Constantine’s Equivalence
- Chapter 31 - Coupling Versus Decoupling
- Conclusion
Foreword
- Larry Constantine:
- Complexity of code depends on how it is organized into parts, on how coupled those parts are with each other, and on how cohesive the parts are in themselves.
- Coupling and cohesion are simply measures of complexity.
Preface
- SW design is an exercise in human relationships.
- Why do we go down the rabbit hole of cleaning code to the exclusion of work that would help our users?
- Design some time around when you can take advantage of the design:
- This requires taste, negotiation and judgement.
- Taste and judgement is an inevitable weakness.
Introduction
- How much and when to do sw design.
Part I - Tidyings
- Tidyings are a subset of refactoring that nobody could possibly hate on.
Chapter 1 - Guard Clauses
- Make code easier to read.
- A routine with 7 or 8 guard clauses is not easier to read.
Chapter 2 - Dead Code
- Delete it.
- If you suspect code isn’t used, pre-tidy it by logging its use.
Chapter 3 - Normalize Symmetries
- As a reader, you expect that difference means difference.
Chapter 4 - New Interface, Old Implementation
- Implement the interface you wish you could call and call it.
- Creating a pass-through interface is the microscale essence of SW design.
Chapter 5 - Reading Order
- No single ordering of elements is perfect.
Chapter 6 - Cohesion Order
- Reorder code so the elements that change together are adjacent.
- Sometimes better cohesion helps you live with the coupling.
Chapter 7 - Move Declaration and Initialization Together
- Is it easier to read if each var is declared and initialized just before it is used, or if they are all declared and initialized together at the top?
Chapter 8 - Explaining Variables
- Extract subexpressions into named variables.
Chapter 9 - Explaining Constants
- I agree with the principle, disagree with the example (404 vs HTTP.NOT_FOUND).
Chapter 10 - Explicit Parameters
- A map with keys is not explicit enough.
- Clojure?
Chapter 11 - Chunk Statements
- Put a blank line between parts of a routine.
- Done well, sw design enables sw design that enables change.
Chapter 12 - Extract Helper
- New interfaces emerge when we’re ready to think more abstractly, to add words to our design vocabulary.
Chapter 13 - One Pile
- Sometimes because of how small pieces interact, the code is harder to understand:
- Inline as much code as you need and tidy from there.
Chapter 14 - Explaining Comments
- Write down what isn’t obvious from the code:
- Keep other people’s perspective in mind.
Part II - Managing
- Tidying is geek self-care.
- Just because you can tidy doesn’t mean you should tidy.
Chapter 16 - Separate Tidying
- Tidying go in their PRs, with as few tidying per PR as possible:
- If you are comfortable with tidying, experiment with not requiring PRs for tidying.
Chapter 17 - Chaining
- Stick to tiny tidying steps.
Chapter 18 - Batch Sizes
- Tidying is not looking towards a far-ahead future, but the immediate need.
- Reduce the cost of reviews (for tidying).
Chapter 19 - Rhythm
- More than one hour tidying at a time before making any behavioral changes, likely means you have lost track of the minimum set of structural changes needed.
- From Pareto rules, most changes will end up in a tidied up area.
Chapter 20 - Getting Untangled
- If you end up with a mess of tidyings and changes all tangled together, discard them and start over, tidying first:
- Is more works.
- Leaves a coherent chain of commits.
- You might see something new.
Chapter 21 - First, After, Later, Never
- When to tidy?
- Never:
- If you are never ever going to touch the code again.
- Very unlikely.
- Later:
- If you don’t have enough time to do your work.
- Create a list of tidyings and work on them when you don’t have the energy or time to start something big.
- Tidying later is a learning tool.
- Feels good: don’t underestimate how much better you are as a programmer when you are happy.
- After:
- If you are going to change the same are again soon.
- It is cheaper to do now.
- Is proportional to the cost of the behavioral change.
- First:
- If the change is easier after the tidying.
- If it helps you understand the code.
- Never:
- Be wary of tidying becoming an end in itself.
Part III - Theory
- Understanding theory optimizes application.
- If we disagree in principle, and we can discuss our principles, then we have a chance to agree sooner.
Chapter 22 - Beneficially Relating Elements
- SW designers can only:
- Create and delete elements.
- Create and delete relationships.
- Increase the benefit of a relationship.
- Structure of a system:
- Element hierarchy.
- Relationship between elements.
- Benefits created by those relationships.
Chapter 23 - Structure and Behaviour
- SW creates value in two ways:
- What it does today (== behaviour).
- The possibility of new things we can make it do tomorrow (== optionality).
- The mere presence of a system behaving a certain way changes the desire for how the system should behave (Heisenberg’s uncertainty principle).
- The more volatile the env is, the more valuable options become.
- A reason for option value to reduce is if exercising an option takes a month instead of a day.
- SW structure creates options.
- We can’t really tell if we have invested or invested enough in SW structure.
Chapter 24 - Economics: Time Value and Optionality
- When geeky imperatives clash with money imperatives, money wins.
- Nature of money:
- A dollar today is worth more than a dollar tomorrow, so earn sooner and spend later.
- In a chaotic situation, options are better than things, so create options in the face of uncertainty.
Chapter 25 - A Dollar Today > A Dollar Tomorrow
- The time value of money encourages tidy after over tidy first.
Chapter 26 - Options
- Economic value of a SW system is the sum of the discounted future cash flows:
- We create value when we change those flows:
- Earning more money, sooner, and with greater likelihood.
- Spending less money, later, and with less likelihood.
- We create value when we change those flows:
Chapter 27 - Options Versus Cash Flows
- Tidying first may make economic sense in spite of discounted cash flows if the value of the options created is greater than the value lost by spending money sooner and with certainty:
- We are firmly in the last of judgement here.
Chapter 28 - Reversible Structure Changes
- There is great value in reviewing, double-checking irreversible decisions. The pace should b slow and deliberate.
- Most SW design decisions are easily reversible, hence there is little value to avoiding mistakes, hence we shouldn’t invest much in doing so.
- Code reviews should distinguish between reversible and irreversible changes, and the investment should be accordingly.
- Make decisions reversible.
Chapter 29 - Coupling
- If two elements are coupled with respect to a change that never happens, then they aren’t coupled in a way that should concern us.
- Analyzing coupling requires knowing what changes have happened and/or likely to happen.
- Cascading coupling are the bigger issue.
- Coupling drives the cost of sw.
Chapter 30 - Constantine’s Equivalence
- The most expensive behaviour changes cost together far more than all the least expensive behaviour changes.
- Constantine’s equivalence:
- cost(sw) ~= cost(changes) ~= cost(big changes) ~= coupling.
- To reduce the cost of SW, we must reduce coupling.
Chapter 31 - Coupling Versus Decoupling
- Coupling often isn’t obvious until you step on it.
- The more you reduce coupling for one class of changes, the greater the coupling becomes for other classes of changes.
Conclusion
- Make SW design an ordinary, balanced part of development.
- Tidy first? Likely yes. Just enough. You are worth it.